results matching ""
No results matching ""
- 1.2.Overview
1.2.Overview
¶ 1.2.1. HTML code
Only need reference two Javascript Libraries in HTML:
<script src="https://TML.ink/X.tml"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/socket.io/2.1.1/socket.io.js"></script>
The first line reference the client function library. This's a dynamic library includes many dynamic components and CDN resources, so don't save to local. For new features, strongly recommend above address which comes with the latest version of X-Server, many new components, enhanced performance, and more. The second line is using third-party socket library from CDN.
¶ 1.2.2. Javascript code
§ 1.2.2.1. Create a client instance
tim = TML('wss://x.TML.ink'); //Create a client instance
If you connect multiple servers at the same time, you can create multiple client instances. Connect a server,Load authentication module, Provide offline login codes and method, logout codes and method, multi-server login, automatic login, user cache and other functions of authentication. If you don't need these features, you should not execute this line of code.
§ 1.2.2.2. Using eXtend feature
tim.X('feature name').xxx()
Feature name can be defined by yourself, starting with lowercase letter and only letters without numbers & symbols.
.xxx() should be Get(), Set(), New(), Del(). These are all asynchronous methods, so every asynchronous method needs to be followed by "then" or add "await" in front.
console.log(await tim.X('feature name').Get());//add "await" in front
Above codes can also be written as follow:
tim.X('feature name').Get().then(data => console.log(data)); //followed by "then"
§ 1.2.2.3. Sign Up a new user
tim.X('user').New({
email: "test email",
password: "test"
});
§ 1.2.2.4. Login code
tim.Login({
email: "test email",
password: "test"
});
Use local cache to automatically login can be written as follow:
tim.Login();
§ 1.2.2.5. Logout code
tim.Logout();
§ 1.2.2.6. Monitor user registration
tim.X('user').on('add', function(msg){
console.log(msg);
});
Listen for real-time messages registered by users. This will be a mass message to update other users' interfaces without refreshing.
§ 1.2.2.7. Get example
console.log(await tim.X('user').Get());//Get top 10 users
//Get top 50 users
console.log(await tim.X('user').Get({
$max: 50
}))
//Get the user whose email is 'a'
console.log(await tim.X('user').Get({
email: 'a'
}))
Above codes are equivalent to:
tim.X('user').Get().then(data => console.log(data));//Get top 10 users
//Get top 50 users
tim.X('user').Get({
$max: 50
}).then(data => console.log(data));
//Get the user whose email is 'a'
tim.X('user').Get({
email: 'a'
}).then(data => console.log(data));
¶ 1.2.3. Operators of Get Method
§ 1.2.3.1. Equals a Specified Value
Specifies equality condition. The $eq operator matches documents where the value of a field equals the specified value. The following example queries the user collection to select all documents where the value of the email field equals a:
tim.X('user').Get({
email: 'a'
}).then(data => console.log(data));
§ 1.2.3.2. $max
The $max stage has the following prototype form:
{ $max: <positive integer> }
$max takes a positive integer that specifies the maximum number of documents to pass along. Consider the following example:
tim.X('user').Get({
$max: 50
}).then(data => console.log(data));
This operation returns only the first 50 documents. $max has no effect on the content of the documents it passes. Set $max to 0 will get the counting faster.
§ 1.2.3.3. $sort
Specifies the order in which the returns matching documents. You can apply $sort to sort by and a value of 1 or -1 to specify an ascending or descending sort respectively before retrieving any documents from the database. The following sample document specifies a descending sort by the _time field and will returns the 10 newest documents:
tim.X('user').Get({
$max: 10,
$sort: {
_time: -1
}
}).then(data => console.log(data));
This operation use $max in conjunction with $sort to return the first (in terms of the sort order) documents.
§ 1.2.3.4. lessThan
$lt selects the documents where the value of the field is less than (i.e.
tim.X('user').Get({
price: {
$lt: 9.9
}
});
¶ 1.2.4. Catch an exception
Use try...catch statement marks a block of statements to try, and specifies a response, should an exception be thrown.
§ 1.2.4.1. Asynchronous catch
For example: Login
tim.Login({
email: "test email",
password: "test"
}).then(function(data) {
console.log(data);
})
.catch(function(err) {
console.log(err);
console.log(err.message);
})
For example: Get
tim.X('user').Get()
.then(function(data) {
console.log(data);
})
.catch(function(err) {
console.log(err);
console.log(err.message);
})
§ 1.2.4.2. Synchronous catch
For example: Login
try {
await tim.Login({
email: "test email",
password: "test"
});
} catch (err) {
console.log(err);
console.log(err.message);
}
For example: Get
try {
await tim.X('user').Get();
} catch (err) {
console.log(err);
console.log(err.message);
}
To Be Continued
This page is just an unfinished draft. The author is writing now. Welcome to donate, cheer for the author.
小礼物刷一波,打赏作者
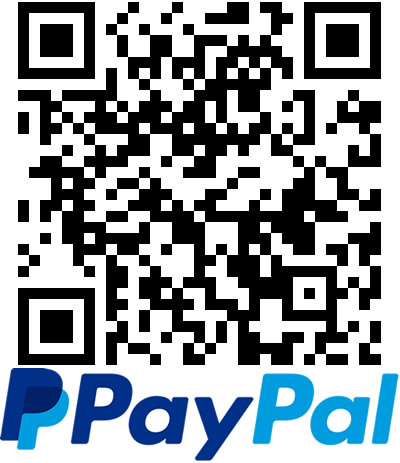
Paypal Donate
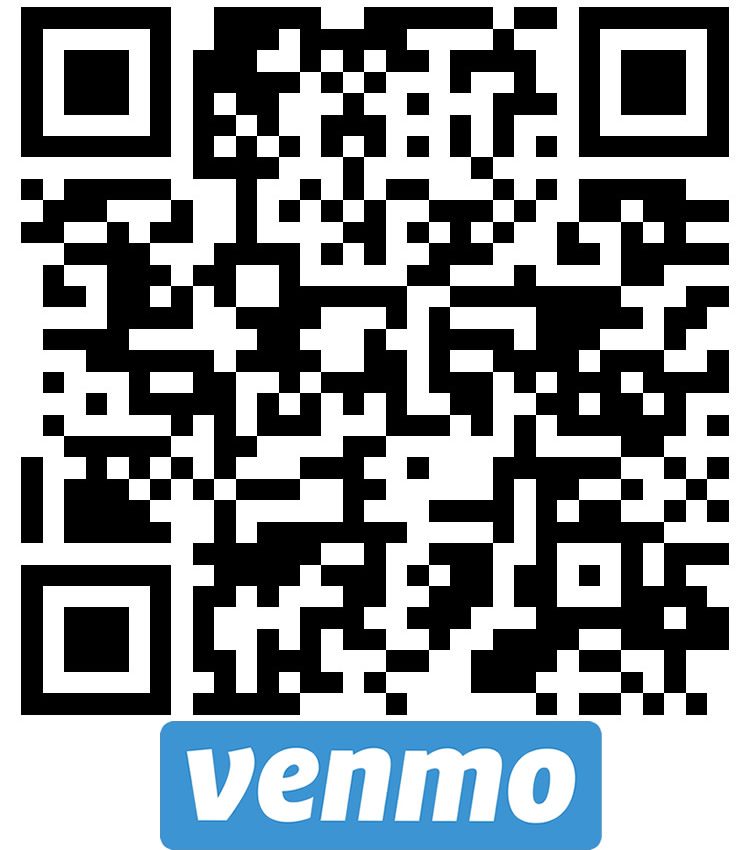
Venmo Donate
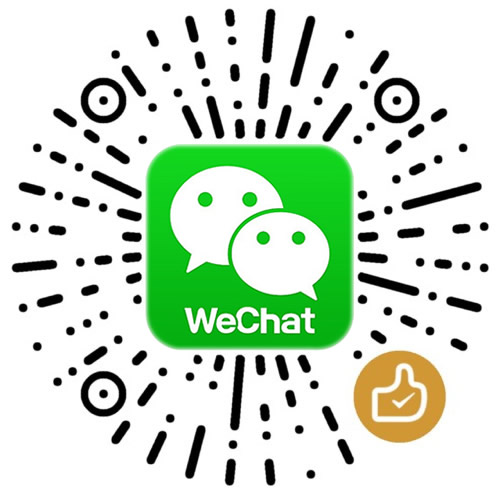
WeChat微信打赏
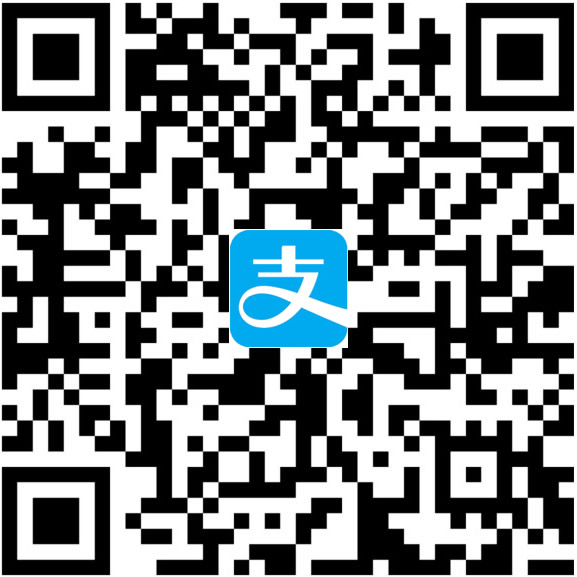
Alipay支付宝打赏