results matching ""
No results matching ""
4.3.Log In
This page provides examples of login operations.
¶ 4.3.1. Prerequisites
See the Sign Up for more information.
§ 4.3.1.1. Dependent Libraries
Only need reference two Javascript Libraries in HTML:
<script src="https://TML.ink/X.tml"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/socket.io/2.1.1/socket.io.js"></script>
The first line reference the client function library. This's a dynamic library includes many dynamic components and CDN resources, so don't save to local. The second line is using third-party socket library from CDN.
§ 4.3.1.2. Create a client instance
tim = TML('wss://x.TML.ink'); //Create a client instance
If you connect multiple servers at the same time, you can create multiple client instances. Connect a server, Load authentication module, Provide offline login codes and method, logout codes and method, multi-server login, automatic login, user cache and other functions of authentication. If you don't need these features, you should not execute this line of code.
§ 4.3.1.3. Log In code
tim.Login({
email: "contact@TML.ink",
password: "123"
});
Use local cache to automatically login can be written as follow:
tim.Login();
§ 4.3.1.4. Logout code
tim.Logout();
§ 4.3.1.5. Get user example
console.log(await tim.X('user').Get());//Get top 10 users
//Get top 50 users
console.log(await tim.X('user').Get({
$max: 50
}))
//Get the user whose email is 'a'
console.log(await tim.X('user').Get({
email: 'contact@TML.ink'
}))
Above codes are equivalent to:
tim.X('user').Get().then(data => console.log(data));//Get top 10 users
//Get top 50 users
tim.X('user').Get({
$max: 50
}).then(data => console.log(data));
//Get the user whose email is 'a'
tim.X('user').Get({
email: 'contact@TML.ink'
}).then(data => console.log(data));
¶ 4.3.2. Catch Log In's exception
Use try...catch statement marks a block of statements to try, and specifies a response, should an exception be thrown.
§ 4.3.2.1. Asynchronous catch
tim.Login({
email: "contact@TML.ink",
password: "123"
}).then(function(rs) {
console.log(rs);
})
.catch(function(err) {
console.log(err);
console.log(err.message);
})
§ 4.3.2.2. Synchronous catch
try {
await tim.Login({
email: "contact@TML.ink",
password: "123"
});
} catch (err) {
console.log(err);
console.log(err.message);
}
¶ 4.3.3. Complete codes of Log In
<HTML>
<h3>Log In</h3>
<p>Email:<input type="text" placeholder="Input your email..."></p>
<p>Password:<input type="password" placeholder="Input your password..."></p>
<p><input type="button" value="Log In"></p>
<p>Test email:contact@TML.ink</p>
<p>Test password:123</p>
<script src="https://TML.ink/X.tml"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/socket.io/2.1.1/socket.io.js"></script>
<script type="text/JavaScript">
tim = TML('wss://x.TML.ink');
$input[2].onclick=e=>{
if(($input[0].value.length<3)||($input[1].value.length<3)){
alert("Please enter the correct email and password");
}else{
tim.Login({
email: $input[0].value,
password: $input[1].value
}).then(function(data) {
alert("Success!");
})
.catch(function(err) {
alert(err.message);
});
}
}
</script>
</HTML>
Run above code in browser: https://Doc.TML.ink/examples/login_code.html
How to run above code in your local computer?View the last article in this chapter.
小礼物刷一波,打赏作者
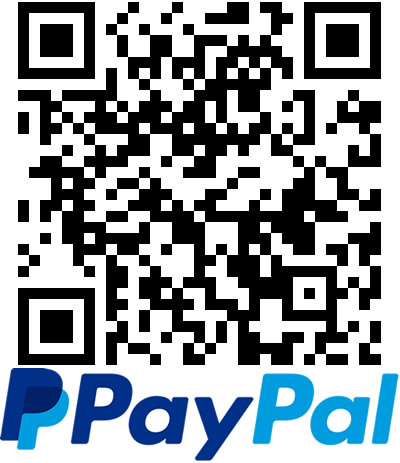
Paypal Donate
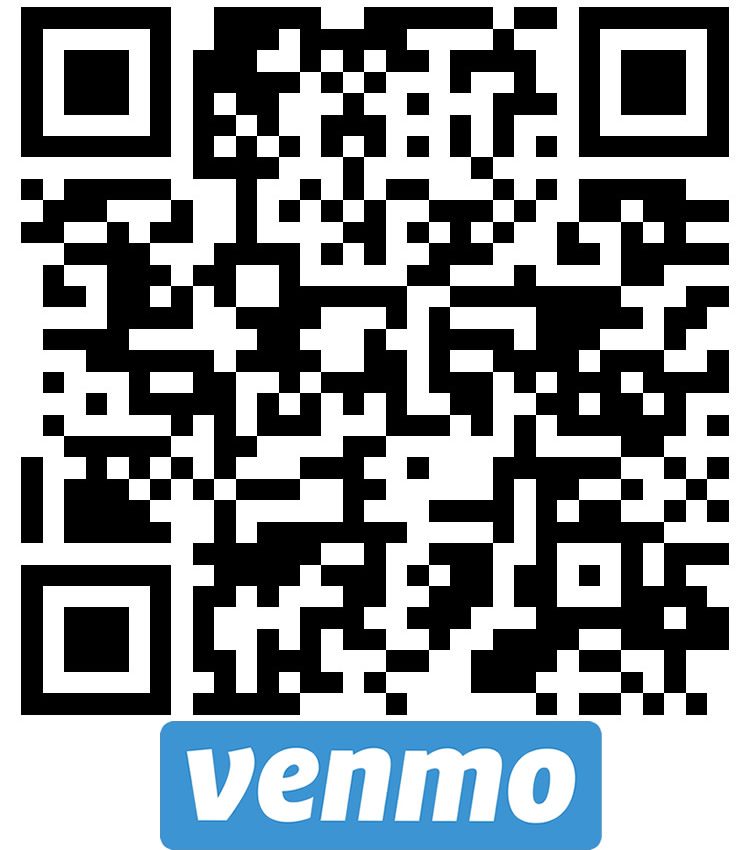
Venmo Donate
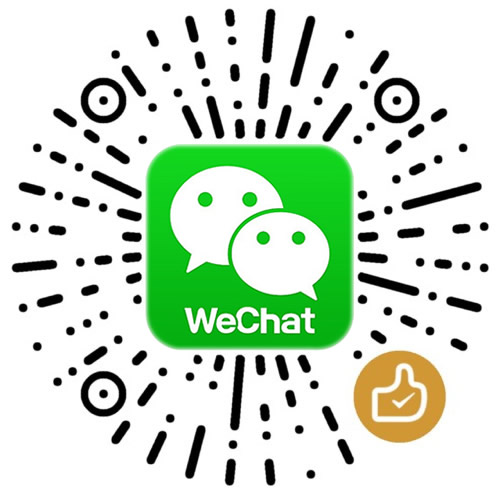
WeChat微信打赏
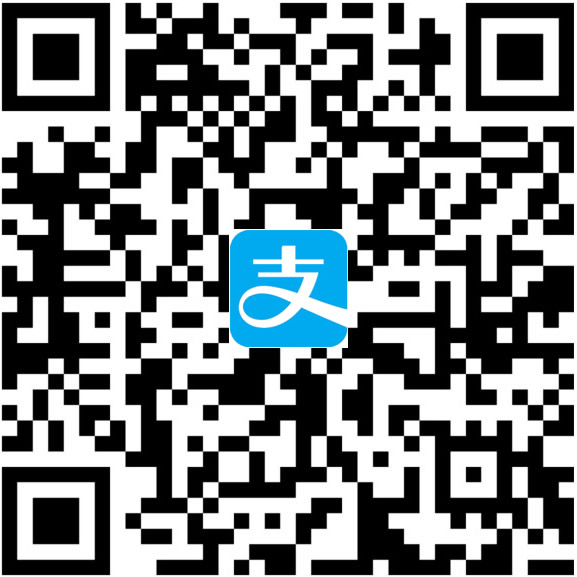
Alipay支付宝打赏