results matching ""
No results matching ""
4.1.Sign Up
¶ 4.1.1. Prerequisites
This tutorial requires you to be connected to the following. (For more information on connecting a server, see Overview.)
§ 4.1.1.1. Dependent Libraries
Only need reference two Javascript Libraries in HTML:
<script src="https://TML.ink/X.tml"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/socket.io/2.1.1/socket.io.js"></script>
The first line reference the client function library. This's a dynamic library includes many dynamic components and CDN resources, so don't save to local. The second line is using third-party socket library from CDN.
§ 4.1.1.2. Create a client instance
tim = TML('wss://x.TML.ink'); //Create a client instance
If you connect multiple servers at the same time, you can create multiple client instances.
Connect a server, Load authentication module, Provide offline login codes and method, logout codes and method, multi-server login, automatic login, user cache and other functions of authentication. If you don't need these features, you should not execute this line of code.
§ 4.1.1.3. Sign Up a new user
tim.X('user').New({
email: "contact@TML.ink",
password: "123"
});
§ 4.1.1.4. Monitor user registration
tim.X('user').on('add', function(msg){
console.log(msg);
});
Listen for real-time messages registered by users. This will be a mass message to update other users' interfaces without refreshing.
¶ 4.1.2. Catch Sign Up's exception
Use try...catch statement marks a block of statements to try, and specifies a response, should an exception be thrown.
§ 4.1.2.1. Asynchronous catch
tim.X('user').New({
email: "contact@TML.ink",
password: "123"
}).then(function(data) {
console.log(data);
})
.catch(function(err) {
console.log(err);
});
§ 4.1.2.2. Synchronous catch
try {
await tim.X('user').New({
email: "contact@TML.ink",
password: "123"
});
} catch (err) {
console.log(err);
};
¶ 4.1.3. Complete codes of Sign Up
<HTML>
<h3>Sign Up</h3>
<p>Email:<input type="text" placeholder="Input your email..."></p>
<p>Password:<input type="password" placeholder="Input your password..."></p>
<p><input type="button" value="Sign Up"></p>
<script src="https://TML.ink/X.tml"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/socket.io/2.1.1/socket.io.js"></script>
<script type="text/JavaScript">
tim = TML('wss://x.TML.ink');
$input[2].onclick=e=>{
if(($input[0].value.length<3)||($input[1].value.length<3)){
alert("Your email and password must be longer than three characters.");
}else{
tim.X('user').New({
email: $input[0].value,
password: $input[1].value
}).then(function(data) {
alert("Success! Your id is "+data._id);
})
.catch(function(err) {
alert(err);
});
}
$input[0].value="";
}
</script>
</HTML>
Run above code in browser: https://Doc.TML.ink/examples/signup_code.html
How to run above code in your local computer?View the last article in this chapter.
小礼物刷一波,打赏作者
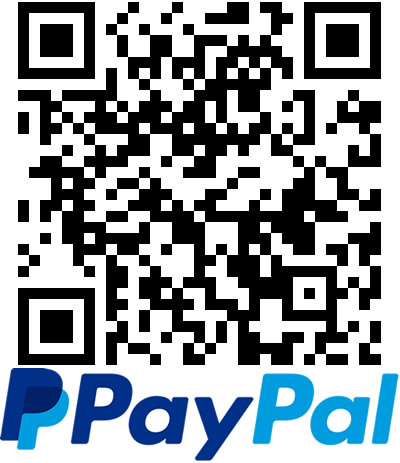
Paypal Donate
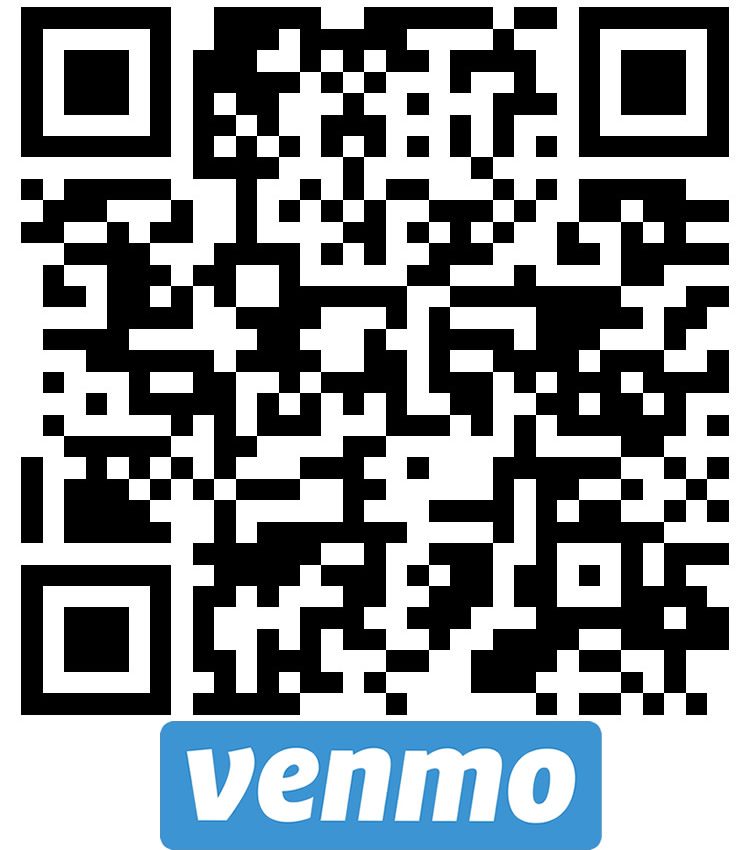
Venmo Donate
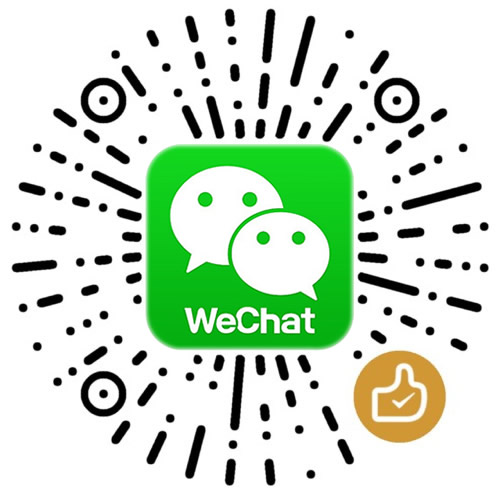
WeChat微信打赏
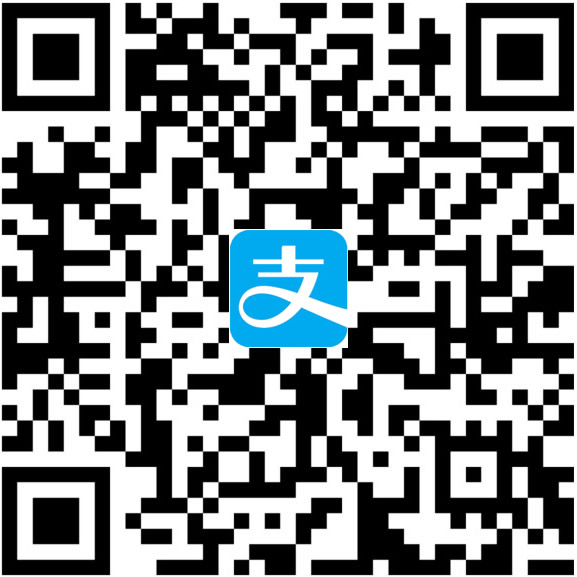
Alipay支付宝打赏