results matching ""
No results matching ""
2.3.Element Query Operators
¶ 2.3.1. Element Operators List
Name | Description |
---|---|
$exists | Matches documents that have the specified field. |
$type | Selects documents if a field is of the specified type. |
¶ 2.3.2. $exists
§ 2.3.2.1. Definition
Syntax: { field: { $exists:
When
§ 2.3.2.2. Example of Exists and Not Equal To
Consider the following example:
tim.X("inventory").Get( { qty: { $exists: true, $nin: [ 5, 15 ] } } )
This query will select all documents in the inventory collection where the qty field exists and its value does not equal 5 or 15.
§ 2.3.2.3. Null Values
The following examples uses a collection named records with the following documents:
{ a: 5, b: 5, c: null }
{ a: 3, b: null, c: 8 }
{ a: null, b: 3, c: 9 }
{ a: 1, b: 2, c: 3 }
{ a: 2, c: 5 }
{ a: 3, b: 2 }
{ a: 4 }
{ b: 2, c: 4 }
{ b: 2 }
{ c: 6 }
§ 2.3.2.4. $exists: true
The following query specifies the query predicate a: { $exists: true }:
tim.X("inventory").Get( { a: { $exists: true } } ) The results consist of those documents that contain the field a, including the document whose field a contains a null value:
{ a: 5, b: 5, c: null }
{ a: 3, b: null, c: 8 }
{ a: null, b: 3, c: 9 }
{ a: 1, b: 2, c: 3 }
{ a: 2, c: 5 }
{ a: 3, b: 2 }
{ a: 4 }
§ 2.3.2.5. $exists: false
The following query specifies the query predicate b: { $exists: false }:
tim.X("inventory").Get( { b: { $exists: false } } ) The results consist of those documents that do not contain the field b:
{ a: 2, c: 5 }
{ a: 4 }
{ c: 6 }
¶ 2.3.3. $type
§ 2.3.3.1. Definition
$type selects the documents where the value of the field is an instance of the specified JSON type(s). Querying by data type is useful when dealing with highly unstructured data where data types are not predictable.
A $type expression for a single JSON type has the following syntax:
{ field: { $type: <JSON type> } }
You can specify either the number or alias for the JSON type
The $type expression can also accept an array of JSON types and has the following syntax:
{ field: { $type: [ <JSON type1> , <JSON type2>, ... ] } }
The above query will match documents where the field value is any of the listed types. The types specified in the array can be either numeric or string aliases.
See Querying by Multiple Data Type for an example.
Available Types describes the JSON types and their corresponding numeric and string aliases.
If you wish to obtain the JSON type returned by an operator expression rather than filtering documents by their JSON type, use the $type aggregation operator.
§ 2.3.3.2. Behavior
$type returns documents where the JSON type of the field matches the JSON type passed to $type.
$type now works with arrays in the same way it works with other JSON types. Previous versions only matched documents where the field contained a nested array.
§ 2.3.3.3. Available Types
$type operator accepts string aliases for the JSON types in addition to the numbers corresponding to the JSON types. Previous versions only accepted the numbers corresponding to the JSON type.
Type | Number | Alias | Notes |
---|---|---|---|
Double | 1 | “double” | |
String | 2 | “string” | |
Object | 3 | “object” | |
Array | 4 | “array” | |
Binary data | 5 | “binData” | |
Undefined | 6 | “undefined” | Deprecated. |
ObjectId | 7 | “objectId” | |
Boolean | 8 | “bool” | |
Date | 9 | “date” | |
Null | 10 | “null” | |
Regular Expression | 11 | “regex” | |
DBPointer | 12 | “dbPointer” | Deprecated. |
JavaScript | 13 | “javascript” | |
Symbol | 14 | “symbol” | Deprecated. |
JavaScript (with scope) | 15 | “javascriptWithScope” | |
32-bit integer | 16 | “int” | |
Timestamp | 17 | “timestamp” | |
64-bit integer | 18 | “long” | |
Decimal128 | 19 | “decimal” | |
Min key | -1 | “minKey” | |
Max key | 127 | “maxKey” |
$type supports the number alias, which will match against the following JSON types:
double 32-bit integer 64-bit integer decimal See Querying by Data Type
§ 2.3.3.4. MinKey and MaxKey
MinKey and MaxKey are used in comparison operations and exist primarily for internal use. For all possible JSON element values, MinKey will always be the smallest value while MaxKey will always be the greatest value.
Querying for minKey or maxKey with $type will only return fields that match the special MinKey or MaxKey values.
Suppose that the data collection has two documents with MinKey and MaxKey:
{ "\_id" : 1, x : { "$minKey" : 1 } }
{ "\_id" : 2, y : { "$maxKey" : 1 } }
The following query will return the document with _id: 1:
tim.X("inventory").Get( { x: { $type: "minKey" } } )
The following query will return the document with _id: 2:
tim.X("inventory").Get( { y: { $type: "maxKey" } } )
§ 2.3.3.5. Example of Querying by Data Type
The addressBook contains addresses and zipcodes, where zipCode has string, int, double, and long values:
tim.X("inventory").New(
[
{ "\_id" : 1, address : "2030 Martian Way", zipCode : "90698345" },
{ "\_id" : 2, address: "156 Lunar Place", zipCode : 43339374 },
{ "\_id" : 3, address : "2324 Pluto Place", zipCode: NumberLong(3921412) },
{ "\_id" : 4, address : "55 Saturn Ring" , zipCode : NumberInt(88602117) }
]
)
The following queries return all documents where zipCode is the JSON type string:
tim.X("inventory").Get( { "zipCode" : { $type : 2 } } );
tim.X("inventory").Get( { "zipCode" : { $type : "string" } } );
These queries return:
{ "\_id" : 1, "address" : "2030 Martian Way", "zipCode" : "90698345" }
The following queries return all documents where zipCode is the JSON type double:
tim.X("inventory").Get( { "zipCode" : { $type : 1 } } )
tim.X("inventory").Get( { "zipCode" : { $type : "double" } } )
These queries return:
{ "\_id" : 2, "address" : "156 Lunar Place", "zip" : 43339374 }
The following query uses the number alias to return documents where zipCode is the JSON type double, int, or long:
tim.X("inventory").Get( { "zipCode" : { $type : "number" } } )
These queries return:
{ "\_id" : 2, address : "156 Lunar Place", zipCode : 43339374 }
{ "\_id" : 3, address : "2324 Pluto Place", zipCode: NumberLong(3921412) }
{ "\_id" : 4, address : "55 Saturn Ring" , zipCode : 88602117 }
§ 2.3.3.6. Querying by Multiple Data Type
The grades collection contains names and averages, where classAverage has string, int, and double values:
tim.X("inventory").New(
[
{ "\_id" : 1, name : "Alice King" , classAverage : 87.333333333333333 },
{ "\_id" : 2, name : "Bob Jenkins", classAverage : "83.52" },
{ "\_id" : 3, name : "Cathy Hart", classAverage: "94.06" },
{ "\_id" : 4, name : "Drew Williams" , classAverage : 93 }
]
)
The following queries return all documents where classAverage is the JSON type string or double. The first query uses numeric aliases while the second query uses string aliases.
tim.X("inventory").Get( { "classAverage" : { $type : [ 2 , 1 ] } } );
tim.X("inventory").Get( { "classAverage" : { $type : [ "string" , "double" ] } } );
These queries return the following documents:
{ "\_id" : 1, name : "Alice King" , classAverage : 87.333333333333333 }
{ "\_id" : 2, name : "Bob Jenkins", classAverage : "83.52" }
{ "\_id" : 3, name : "Cathy Hart", classAverage: "94.06" }
§ 2.3.3.7. Querying by MinKey and MaxKey
The restaurants collection uses minKey for any grade that is a failing grade:
{
"\_id": 1,
"address": {
"building": "230",
"coord": [ -73.996089, 40.675018 ],
"street": "Huntington St",
"zipcode": "11231"
},
"borough": "Brooklyn",
"cuisine": "Bakery",
"grades": [
{ "date": new Date(1393804800000), "grade": "C", "score": 15 },
{ "date": new Date(1378857600000), "grade": "C", "score": 16 },
{ "date": new Date(1358985600000), "grade": MinKey(), "score": 30 },
{ "date": new Date(1322006400000), "grade": "C", "score": 15 }
],
"name": "Dirty Dan's Donuts",
"restaurant\_id": "30075445"
}
And maxKey for any grade that is the highest passing grade:
{
"\_id": 2,
"address": {
"building": "1166",
"coord": [ -73.955184, 40.738589 ],
"street": "Manhattan Ave",
"zipcode": "11222"
},
"borough": "Brooklyn",
"cuisine": "Bakery",
"grades": [
{ "date": new Date(1393804800000), "grade": MaxKey(), "score": 2 },
{ "date": new Date(1378857600000), "grade": "B", "score": 6 },
{ "date": new Date(1358985600000), "grade": MaxKey(), "score": 3 },
{ "date": new Date(1322006400000), "grade": "B", "score": 5 }
],
"name": "Dainty Daisey's Donuts",
"restaurant\_id": "30075449"
}
The following query returns any restaurant whose grades.grade field contains minKey:
tim.X("inventory").Get(
{ "grades.grade" : { $type : "minKey" } }
)
This returns
{
"\_id" : 1,
"address" : {
"building" : "230",
"coord" : [ -73.996089, 40.675018 ],
"street" : "Huntington St",
"zipcode" : "11231"
},
"borough" : "Brooklyn",
"cuisine" : "Bakery",
"grades" : [
{ "date" : ISODate("2014-03-03T00:00:00Z"), "grade" : "C", "score" : 15 },
{ "date" : ISODate("2013-09-11T00:00:00Z"), "grade" : "C", "score" : 16 },
{ "date" : ISODate("2013-01-24T00:00:00Z"), "grade" : { "$minKey" : 1 }, "score" : 30 },
{ "date" : ISODate("2011-11-23T00:00:00Z"), "grade" : "C", "score" : 15 }
],
"name" : "Dirty Dan's Donuts",
"restaurant\_id" : "30075445"
}
The following query returns any restaurant whose grades.grade field contains maxKey:
tim.X("inventory").Get(
{ "grades.grade" : { $type : "maxKey" } }
)
This returns
{
"\_id" : 2,
"address" : {
"building" : "1166",
"coord" : [ -73.955184, 40.738589 ],
"street" : "Manhattan Ave",
"zipcode" : "11222"
},
"borough" : "Brooklyn",
"cuisine" : "Bakery",
"grades" : [
{ "date" : ISODate("2014-03-03T00:00:00Z"), "grade" : { "$maxKey" : 1 }, "score" : 2 },
{ "date" : ISODate("2013-09-11T00:00:00Z"), "grade" : "B", "score" : 6 },
{ "date" : ISODate("2013-01-24T00:00:00Z"), "grade" : { "$maxKey" : 1 }, "score" : 3 },
{ "date" : ISODate("2011-11-23T00:00:00Z"), "grade" : "B", "score" : 5 }
],
"name" : "Dainty Daisey's Donuts",
"restaurant\_id" : "30075449"
}
§ 2.3.3.8. Querying by Array Type
A collection named SensorReading contains the following documents:
{
"\_id": 1,
"readings": [
25,
23,
[ "Warn: High Temp!", 55 ],
[ "ERROR: SYSTEM SHUTDOWN!", 66 ]
]
},
{
"\_id": 2,
"readings": [
25,
25,
24,
23
]
},
{
"\_id": 3,
"readings": [
22,
24,
[]
]
},
{
"\_id": 4,
"readings": []
},
{
"\_id": 5,
"readings": 24
}
The following query returns any document in which the readings field is an array, empty or non-empty.
tim.X("inventory").Get( { "readings" : { $type: "array" } } )
The above query returns the following documents:
{
"\_id": 1,
"readings": [
25,
23,
[ "Warn: High Temp!", 55 ],
[ "ERROR: SYSTEM SHUTDOWN!", 66 ]
]
},
{
"\_id": 2,
"readings": [
25,
25,
24,
23
]
},
{
"\_id": 3,
"readings": [
22,
24,
[]
]
},
{
"\_id": 4,
"readings": []
}
In the documents with _id : 1, _id : 2, _id : 3, and _id : 4, the readings field is an array.
小礼物刷一波,打赏作者
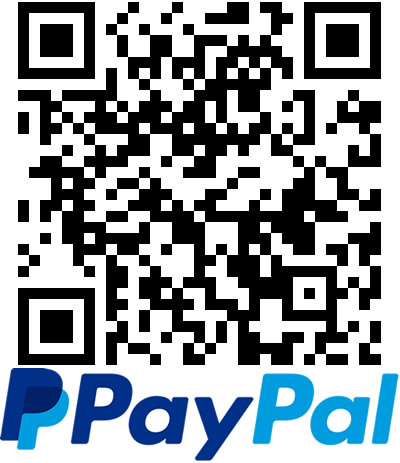
Paypal Donate
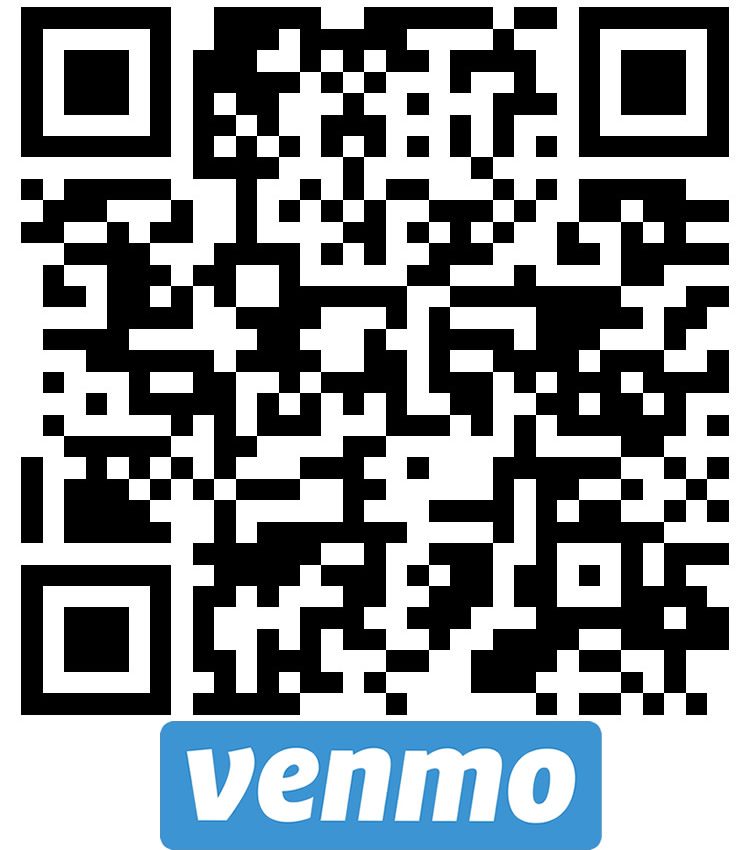
Venmo Donate
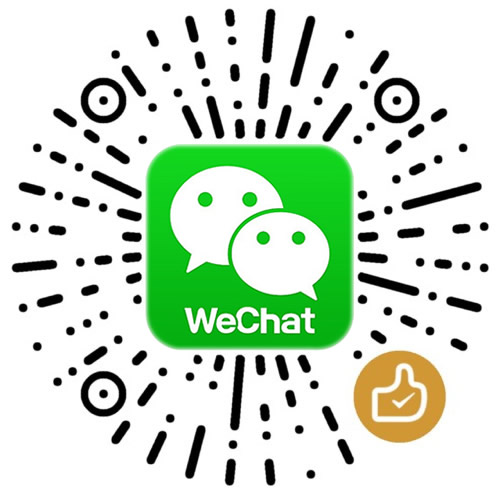
WeChat微信打赏
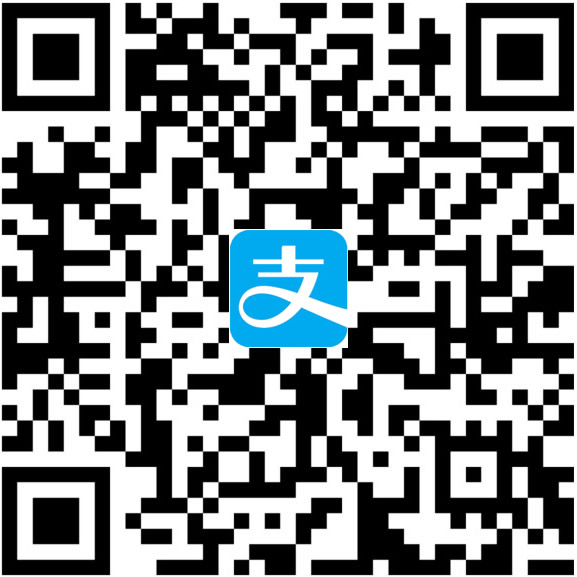
Alipay支付宝打赏