results matching ""
No results matching ""
- 2.4.Evaluation Query Operators
- ¶ 2.4.1. Evaluation Operators List
- ¶ 2.4.2. $expr
- § 2.4.2.1. Behavior
- § 2.4.2.2. Example of Compare Two Fields from A Single Document
- § 2.4.2.3. Using $expr With Conditional Statements
- ¶ 2.4.3. $jsonSchema
- § 2.4.3.1. Behavior
- § 2.4.3.2. Available Keywords
- § 2.4.3.3. Omissions
- § 2.4.3.4. Example of Schema Validation
- ¶ 2.4.4. $mod
- § 2.4.4.1. Example of Use $mod to Select Documents
- § 2.4.4.2. Not Enough Elements Error
- § 2.4.4.3. Array with Single Element
- § 2.4.4.4. Empty Array
- § 2.4.4.5. Too Many Elements Error
- ¶ 2.4.5. $regex
- § 2.4.5.1. $options
- § 2.4.5.2. $regex vs. /pattern/ Syntax
- § 2.4.5.3. Implicit AND Conditions for the Field
- § 2.4.5.4. x and s Options
- § 2.4.5.5. PCRE vs JavaScript
- § 2.4.5.6. Index Use
- § 2.4.5.7. Examples
- § 2.4.5.8. Perform a LIKE Match
- § 2.4.5.9. Perform Case-Insensitive Regular Expression Match
- § 2.4.5.10. Multiline Match for Lines Starting with Specified Pattern
- § 2.4.5.11. Use the . Dot Character to Match New Line
- § 2.4.5.12. Ignore White Spaces in Pattern
- ¶ 2.4.6. $text
- § 2.4.6.1. Behavior of Restrictions
- § 2.4.6.2. $search Field
- § 2.4.6.3. Phrases
- § 2.4.6.4. Negations
- § 2.4.6.5. Match Operation
- § 2.4.6.6. Case Insensitivity
- § 2.4.6.7. Case Sensitive Search Process
- § 2.4.6.8. Diacritic Insensitivity
- § 2.4.6.9. Diacritic Sensitive Search Process
- § 2.4.6.10. Text Score
- § 2.4.6.11. Examples
- § 2.4.6.12. Search for a Single Word
- § 2.4.6.13. Match Any of the Search Terms
- § 2.4.6.14. Search for a Phrase
- § 2.4.6.15. Exclude Documents That Contain a Term
- § 2.4.6.16. Search a Different Language
- § 2.4.6.17. Case and Diacritic Insensitive Search
- § 2.4.6.18. Perform Case Sensitive Search
- § 2.4.6.19. Diacritic Sensitive Search
- ¶ 2.4.7. $where
2.4.Evaluation Query Operators
¶ 2.4.1. Evaluation Operators List
Name | Description |
---|---|
$expr | Allows use of aggregation expressions within the query language. |
$jsonSchema | Validate documents against the given JSON Schema. |
$mod | Performs a modulo operation on the value of a field and selects documents with a specified result. |
$regex | Selects documents where values match a specified regular expression. |
$text | Performs text search. |
$where | Matches documents that satisfy a JavaScript expression. |
¶ 2.4.2. $expr
Allows the use of aggregation expressions within the query language.
$expr has the following syntax:
{ $expr: { <expression> } }
The arguments can be any valid expression.
§ 2.4.2.1. Behavior
$expr can build query expressions that compare fields from the same document in a $match stage.
If the $match stage is part of a $lookup stage, $expr can compare fields using let variables.
$expr does not support multikey indexes.
§ 2.4.2.2. Example of Compare Two Fields from A Single Document
Consider an monthlyBudget collection with the following documents:
{ "\_id" : 1, "category" : "food", "budget": 400, "spent": 450 }
{ "\_id" : 2, "category" : "drinks", "budget": 100, "spent": 150 }
{ "\_id" : 3, "category" : "clothes", "budget": 100, "spent": 50 }
{ "\_id" : 4, "category" : "misc", "budget": 500, "spent": 300 }
{ "\_id" : 5, "category" : "travel", "budget": 200, "spent": 650 }
The following operation uses $expr to find documents where the spent amount exceeds the budget:
tim.X("budget").Get( { $expr: { $gt: [ "$spent" , "$budget" ] } } )
The operation returns the following results:
{ "\_id" : 1, "category" : "food", "budget" : 400, "spent" : 450 }
{ "\_id" : 2, "category" : "drinks", "budget" : 100, "spent" : 150 }
{ "\_id" : 5, "category" : "travel", "budget" : 200, "spent" : 650 }
§ 2.4.2.3. Using $expr With Conditional Statements
Consider a supplies collection with the following documents. If the qty is at least 100, a 50% discount is applied. Otherwise, a 25% discount is applied:
{ "\_id" : 1, "item" : "binder", "qty": 100 , "price": 12 }
{ "\_id" : 2, "item" : "notebook", "qty": 200 , "price": 8 }
{ "\_id" : 3, "item" : "pencil", "qty": 50 , "price": 6 }
{ "\_id" : 4, "item" : "eraser", "qty": 150 , "price": 3 }
The following operation uses $expr with $cond to mimic a conditional statement. It finds documents where the price is less than 5 after the applied discounts.
If the document has a qty value greater than or equal to 100, the query divides the price by 2. Otherwise, it divides the price by 4:
tim.X("budget").Get( {
$expr: {
$lt:[ {
$cond: {
if: { $gte: ["$qty", 100] },
then: { $divide: ["$price", 2] },
else: { $divide: ["$price", 4] }
}
},
5 ] }
} )
The operation returns the following results:
{ "\_id" : 2, "item" : "notebook", "qty": 200 , "price": 8 }
{ "\_id" : 3, "item" : "pencil", "qty": 50 , "price": 6 }
{ "\_id" : 4, "item" : "eraser", "qty": 150 , "price": 3 }
¶ 2.4.3. $jsonSchema
The $jsonSchema operator matches documents that validate against the given JSON Schema.
{ $jsonSchema: <schema> }
§ 2.4.3.1. Behavior
$jsonSchema can be used in a document validator, which enforces that inserted or updated documents are valid against the schema. It can also be used to query for documents with the find command or $match aggregation stage.
§ 2.4.3.2. Available Keywords
Keyword | Type | Definition | Behavior |
---|---|---|---|
JSONType | all types | string alias or array of string aliases | Accepts same string aliases used for the $type operator |
enum | all types | array of values | Enumerates all possible values of the field |
type | all types | string or array of unique strings | Enumerates the possible JSON types of the field. Available types are “object”, “array”, “number”, “boolean”, “string”, and “null”. |
allOf | all types | array of JSON Schema objects | Field must match all specified schemas |
anyOf | all types | array of JSON Schema objects | Field must match at least one of the specified schemas |
oneOf | all types | array of JSON Schema objects | Field must match exactly one of the specified schemas |
not | all types | a JSON Schema object | Field must not match the schema |
multipleOf | numbers | number | Field must be a multiple of this value |
maximum | numbers | number | Indicates the maximum value of the field |
exclusiveMaximum | numbers | boolean | If true and field is a number, maximum is an exclusive maximum. Otherwise, it is an inclusive maximum. |
minimum | numbers | number | Indicates the minimum value of the field |
exclusiveMinimum | numbers | boolean | If true, minimum is an exclusive minimum. Otherwise, it is an inclusive minimum. |
maxLength | strings | integer | Indicates the maximum length of the field |
minLength | strings | integer | Indicates the minimum length of the field |
pattern | strings | string | containing a regex Field must match the regular expression |
maxProperties | objects | integer | Indicates the field’s maximum number of properties |
minProperties | objects | integer | Indicates the field’s minimum number of properties |
required | objects | array of unique strings | Object’s property set must contain all the specified elements in the array |
additionalProperties | objects | boolean or object | If true, additional fields are allowed. If false, they are not. If a valid JSON Schema object is specified, additional fields must validate against the schema. Defaults to true. |
properties | objects | object | A valid JSON Schema where each value is also a valid JSON Schema object |
patternProperties | objects | object | In addition to properties requirements, each property name of this object must be a valid regular expression |
dependencies | objects | object | Describes field or schema dependencies |
additionalItems | arrays | boolean or object | If an object, must be a valid JSON Schema |
items | arrays | object or array | Must be either a valid JSON Schema, or an array of valid JSON Schemas |
maxItems | arrays | integer | Indicates the maximum length of array |
minItems | arrays | integer | Indicates the minimum length of array |
uniqueItems | arrays | boolean | If true, each item in the array must be unique. Otherwise, no uniqueness constraint is enforced. |
title | N/A | string | A descriptive title string with no effect. |
description | N/A | string | A string that describes the schema and has no effect. |
§ 2.4.3.3. Omissions
Hypertext definitions in draft 4 of the JSON Schema spec. The keywords:
$ref
$schema
default
definitions
format
id
The integer type. You must use the JSON type int or long with the JSONType keyword. Hypermedia and linking properties of JSON Schema, including the use of JSON References and JSON Pointers. Unknown keywords.
§ 2.4.3.4. Example of Schema Validation
The following method creates a collection named students and uses the $jsonSchema operator to set multiple rules for the schema design:
tim.X("students", {
validator: {
$jsonSchema: {
JSONType: "object",
required: [ "name", "year", "major", "gpa", "address.city", "address.street" ],
properties: {
name: {
JSONType: "string",
description: "must be a string and is required"
},
gender: {
JSONType: "string",
description: "must be a string and is not required"
},
year: {
JSONType: "int",
minimum: 2017,
maximum: 3017,
exclusiveMaximum: false,
description: "must be an integer in [ 2017, 3017 ] and is required"
},
major: {
enum: [ "Math", "English", "Computer Science", "History", null ],
description: "can only be one of the enum values and is required"
},
gpa: {
JSONType: [ "double" ],
description: "must be a double and is required"
},
"address.city" : {
JSONType: "string",
description: "must be a string and is required"
},
"address.street" : {
JSONType: "string",
description: "must be a string and is required"
}
}
}
}
});
Given the created validator for the collection, the following insert operation will fail because gpa is an integer when the validator requires a double.
tim.X("user").New({
name: "Alice",
year: NumberInt(2019),
major: "History",
gpa: NumberInt(3),
address: {
city: "NYC",
street: "33rd Street"
}
})
The operation returns the following error:
WriteResult({
"nInserted" : 0,
"writeError" : {
"code" : 121,
"errmsg" : "Document failed validation"
}
})
¶ 2.4.4. $mod
Select documents where the value of a field divided by a divisor has the specified remainder (i.e. perform a modulo operation to select documents). To specify a $mod expression, use the following syntax:
{ field: { $mod: [ divisor, remainder ] } }
The $mod operator errors when passed an array with fewer or more elements. In previous versions, if passed an array with one element, the $mod operator uses 0 as the remainder value, and if passed an array with more than two elements, the $mod ignores all but the first two elements. Previous versions do return an error when passed an empty array.
§ 2.4.4.1. Example of Use $mod to Select Documents
Consider a collection inventory with the following documents:
{ "\_id" : 1, "item" : "abc123", "qty" : 0 }
{ "\_id" : 2, "item" : "xyz123", "qty" : 5 }
{ "\_id" : 3, "item" : "ijk123", "qty" : 12 }
Then, the following query selects those documents in the inventory collection where value of the qty field modulo 4 equals 0:
tim.X("inventory").Get( { qty: { $mod: [ 4, 0 ] } } )
The query returns the following documents:
{ "\_id" : 1, "item" : "abc123", "qty" : 0 }
{ "\_id" : 3, "item" : "ijk123", "qty" : 12 }
§ 2.4.4.2. Not Enough Elements Error
The $mod operator errors when passed an array with fewer than two elements.
§ 2.4.4.3. Array with Single Element
The following operation incorrectly passes the $mod operator an array that contains a single element:
tim.X("inventory").Get( { qty: { $mod: [ 4 ] } } )
The statement results in the following error:
error: {
"$err" : "bad query: BadValue malformed mod, not enough elements",
"code" : 16810
}
In previous versions, if passed an array with one element, the $mod operator uses the specified element as the divisor and 0 as the remainder value.
§ 2.4.4.4. Empty Array
The following operation incorrectly passes the $mod operator an empty array:
tim.X("inventory").Get( { qty: { $mod: [ ] } } )
The statement results in the following error:
error: {
"$err" : "bad query: BadValue malformed mod, not enough elements",
"code" : 16810
}
Previous versions returned the following error:
error: { "$err" : "mod can't be 0", "code" : 10073 }
§ 2.4.4.5. Too Many Elements Error
The $mod operator errors when passed an array with more than two elements.
For example, the following operation attempts to use the $mod operator with an array that contains four elements:
error: {
"$err" : "bad query: BadValue malformed mod, too many elements",
"code" : 16810
}
In previous versions, if passed an array with more than two elements, the $mod ignores all but the first two elements.
¶ 2.4.5. $regex
Provides regular expression capabilities for pattern matching strings in queries.
To use $regex, use one of the following syntaxes:
{ <field>: { $regex: /pattern/, $options: '<options>' } }
{ <field>: { $regex: 'pattern', $options: '<options>' } }
{ <field>: { $regex: /pattern/<options> } }
You can also use regular expression objects| (i.e. /pattern/) to specify regular expressions:
{ <field>: /pattern/<options> }
For restrictions on particular syntax use, see $regex vs. /pattern/ Syntax.
§ 2.4.5.1. $options
The following
Option | Description | Syntax | Restrictions | |
---|---|---|---|---|
i | Case insensitivity to match upper and lower cases. For an example, see Perform Case-Insensitive Regular Expression Match. | |||
m | For patterns that include anchors (i.e. ^ for the start, $ for the end), match at the beginning or end of each line for strings | with multiline values. Without this option, these anchors match at beginning or end of the string. For an example, see Multiline Match for Lines Starting with Specified Pattern. If the pattern contains no anchors or if the string value has no newline characters (e.g. \n), the m option has no effect. | ||
x | “Extended” capability to ignore all white space characters in the $regex pattern unless escaped or included in a character class. Additionally, it ignores characters in-between and including an un-escaped hash/pound (#) character and the next new line, so that you may include comments in complicated patterns. This only applies to data characters; white space characters may never appear within special character sequences in a pattern. The x option does not affect the handling of the VT character (i.e. code 11). | Requires $regex with $options syntax | ||
s | Allows the dot character (i.e. .) to match all characters including newline characters. For an example, see Use the . Dot Character to Match New Line. | Requires $regex with $options syntax |
§ 2.4.5.2. $regex vs. /pattern/ Syntax
$in Expressions To include a regular expression in an $in query expression, you can only use JavaScript regular expression objects| (i.e. /pattern/ ). For example:
{ name: { $in: [ /^acme/i, /^ack/ ] } }
You cannot use $regex operator expressions inside an $in.
§ 2.4.5.3. Implicit AND Conditions for the Field
To include a regular expression in a comma-separated list of query conditions for the field, use the $regex operator. For example:
{ name: { $regex: /acme.\*corp/i, $nin: [ 'acmeblahcorp' ] } }
{ name: { $regex: /acme.\*corp/, $options: 'i', $nin: [ 'acmeblahcorp' ] } }
{ name: { $regex: 'acme.\*corp', $options: 'i', $nin: [ 'acmeblahcorp' ] } }
§ 2.4.5.4. x and s Options
To use either the x option or s options, you must use the $regex operator expression with the $options operator. For example, to specify the i and the s options, you must use $options for both:
{ name: { $regex: /acme.\*corp/, $options: "si" } }
{ name: { $regex: 'acme.\*corp', $options: "si" } }
§ 2.4.5.5. PCRE vs JavaScript
To use PCRE supported features in the regex pattern that are unsupported in JavaScript, you must use the $regex operator expression with the pattern as a string. For example, to use (?i) in the pattern to turn case-insensitivity on for the remaining pattern and (?-i) to turn case-sensitivity on for the remaining pattern, you must use the $regex operator with the pattern as a string:
{ name: { $regex: '(?i)a(?-i)cme' } }
§ 2.4.5.6. Index Use
For case sensitive regular expression queries, if an index exists for the field, then X-Server matches the regular expression against the values in the index, which can be faster than a collection scan. Further optimization can occur if the regular expression is a “prefix expression”, which means that all potential matches start with the same string. This allows X-Server to construct a “range” from that prefix and only match against those values from the index that fall within that range.
A regular expression is a “prefix expression” if it starts with a caret (^) or a left anchor (\A), followed by a string of simple symbols. For example, the regex /^abc.*/ will be optimized by matching only against the values from the index that start with abc.
Additionally, while /^a/, /^a.*/, and /^a.*$/ match equivalent strings|, they have different performance characteristics. All of these expressions use an index if an appropriate index exists; however, /^a.*/, and /^a.*$/ are slower. /^a/ can stop scanning after matching the prefix.
Case insensitive regular expression queries generally cannot use indexes effectively. The $regex implementation is not collation-aware and is unable to utilize case-insensitive indexes.
§ 2.4.5.7. Examples
The following examples use a collection products with the following documents:
{ "\_id" : 100, "sku" : "abc123", "description" : "Single line description." }
{ "\_id" : 101, "sku" : "abc789", "description" : "First line\nSecond line" }
{ "\_id" : 102, "sku" : "xyz456", "description" : "Many spaces before line" }
{ "\_id" : 103, "sku" : "xyz789", "description" : "Multiple\nline description" }
§ 2.4.5.8. Perform a LIKE Match
The following example matches all documents where the sku field is like "%789":
tim.X("user").Get( { sku: { $regex: /789$/ } } )
The example is analogous to the following SQL LIKE statement:
SELECT \* FROM products
WHERE sku like "%789";
§ 2.4.5.9. Perform Case-Insensitive Regular Expression Match
The following example uses the i option perform a case-insensitive match for documents with sku value that starts with ABC.
tim.X("user").Get( { sku: { $regex: /^ABC/i } } )
The query matches the following documents:
{ "\_id" : 100, "sku" : "abc123", "description" : "Single line description." }
{ "\_id" : 101, "sku" : "abc789", "description" : "First line\nSecond line" }
§ 2.4.5.10. Multiline Match for Lines Starting with Specified Pattern
The following example uses the m option to match lines starting with the letter S for multiline strings|:
tim.X("user").Get( { description: { $regex: /^S/, $options: 'm' } } )
The query matches the following documents:
{ "\_id" : 100, "sku" : "abc123", "description" : "Single line description." }
{ "\_id" : 101, "sku" : "abc789", "description" : "First line\nSecond line" }
Without the m option, the query would match just the following document:
{ "\_id" : 100, "sku" : "abc123", "description" : "Single line description." }
If the $regex pattern does not contain an anchor, the pattern matches against the string as a whole, as in the following example:
tim.X("user").Get( { description: { $regex: /S/ } } )
Then, the $regex would match both documents:
{ "\_id" : 100, "sku" : "abc123", "description" : "Single line description." }
{ "\_id" : 101, "sku" : "abc789", "description" : "First line\nSecond line" }
§ 2.4.5.11. Use the . Dot Character to Match New Line
The following example uses the s option to allow the dot character (i.e. .) to match all characters including new line as well as the i option to perform a case-insensitive match:
tim.X("user").Get( { description: { $regex: /m.\*line/, $options: 'si' } } )
The query matches the following documents:
{ "\_id" : 102, "sku" : "xyz456", "description" : "Many spaces before line" }
{ "\_id" : 103, "sku" : "xyz789", "description" : "Multiple\nline description" }
Without the s option, the query would have matched only the following document:
{ "\_id" : 102, "sku" : "xyz456", "description" : "Many spaces before line" }
§ 2.4.5.12. Ignore White Spaces in Pattern
The following example uses the x option ignore white spaces and the comments, denoted by the # and ending with the \n in the matching pattern:
var pattern = "abc #category code\n123 #item number"
tim.X("user").Get( { sku: { $regex: pattern, $options: "x" } } )
The query matches the following document:
{ "\_id" : 100, "sku" : "abc123", "description" : "Single line description." }
¶ 2.4.6. $text
$text performs a text search on the content of the fields indexed with a text index. A $text expression has the following syntax:
{
$text:
{
$search: <string>,
$language: <string>,
$caseSensitive: <boolean>,
$diacriticSensitive: <boolean>
}
}
The $text operator accepts a text query document with the following fields:
Field | Type | Description |
---|---|---|
$search | string | A string of terms that X-Server parses and uses to query the text index. X-Server performs a logical OR search of the terms unless specified as a phrase. See Behavior for more information on the field. |
$language | string | Optional. The language that determines the list of stop words for the search and the rules for the stemmer and tokenizer. If not specified, the search uses the default language of the index. For supported languages, see Text Search Languages. If you specify a language value of "none", then the text search uses simple tokenization with no list of stop words and no stemming. |
$caseSensitive | boolean | Optional. A boolean flag to enable or disable case sensitive search. Defaults to false; i.e. the search defers to the case insensitivity of the text index. |
$diacriticSensitive | boolean | Optional. A boolean flag to enable or disable diacritic sensitive search against version 3 text indexes. Defaults to false; i.e. the search defers to the diacritic insensitivity of the text index. Text searches against earlier versions of the text index are inherently diacritic sensitive and cannot be diacritic insensitive. As such, the $diacriticSensitive option has no effect with earlier versions of the text index. |
The $text operator, by default, does not return results sorted in terms of the results’ scores. For more information on sorting by the text search scores, see the Text Score documentation.
§ 2.4.6.1. Behavior of Restrictions
A query can specify, at most, one $text expression. The $text query can not appear in $nor expressions. The $text query can not appear in $elemMatch query expressions or $elemMatch projection expressions. To use a $text query in an $or expression, all clauses in the $or array must be indexed. You cannot use hint() if the query includes a $text query expression. You cannot specify $natural sort order if the query includes a $text expression. You cannot combine the $text expression, which requires a special text index, with a query operator that requires a different type of special index. For example you cannot combine $text expression with the $near operator. Views do not support text search. If using the $text operator in aggregation, the following restrictions also apply.
The $match stage that includes a $text must be the first stage in the pipeline. A text operator can only occur once in the stage. The text operator expression cannot appear in $or or $not expressions. The text search, by default, does not return the matching documents in order of matching scores. Use the $meta aggregation expression in the $sort stage.
§ 2.4.6.2. $search Field
In the $search field, specify a string of words that the text operator parses and uses to query the text index.
The text operator treats most punctuation in the string as delimiters, except a hyphen-minus (-) that negates term or an escaped double quotes \" that specifies a phrase.
§ 2.4.6.3. Phrases
To match on a phrase, as opposed to individual terms, enclose the phrase in escaped double quotes (\"), as in:
"\"ssl certificate\"" If the $search string includes a phrase and individual terms, text search will only match the documents that include the phrase. More specifically, the search performs a logical AND of the phrase with the individual terms in the search string.
For example, passed a $search string:
"\"ssl certificate\" authority key" The $text operator searches for the phrase "ssl certificate" and ("authority" or "key" or "ssl" or "certificate" ).
§ 2.4.6.4. Negations
Prefixing a word with a hyphen-minus (-) negates a word:
The negated word excludes documents that contain the negated word from the result set. When passed a search string that only contains negated words, text search will not match any documents. A hyphenated word, such as pre-market, is not a negation. If used in a hyphenated word, $text operator treats the hyphen-minus (-) as a delimiter. To negate the word market in this instance, include a space between pre and -market, i.e., pre -market. The $text operator adds all negations to the query with the logical AND operator.
§ 2.4.6.5. Match Operation
1. Stop Words
The $text operator ignores language-specific stop words, such as the and and in English.
2. Stemmed Words
For case insensitive and diacritic insensitive text searches, the $text operator matches on the complete stemmed word. So if a document field contains the word blueberry, a search on the term blue will not match. However, blueberry or blueberries will match.
3. Case Sensitive Search and Stemmed Words
For case sensitive search (i.e. $caseSensitive: true), if the suffix stem contains uppercase letters, the $text operator matches on the exact word.
4. Diacritic Sensitive Search and Stemmed Words
For diacritic sensitive search (i.e. $diacriticSensitive: true), if the suffix stem contains the diacritic mark or marks, the $text operator matches on the exact word.
§ 2.4.6.6. Case Insensitivity
The $text operator defaults to the case insensitivity of the text index:
The text index is case insensitive for Latin characters with or without diacritics and characters from non-Latin alphabets, such as the Cyrillic alphabet. See text index for details. Earlier versions of the text index are case insensitive for Latin characters without diacritic marks; i.e. for [A-z]. $caseSensitive Option To support case sensitive search where the text index is case insensitive, specify $caseSensitive: true.
§ 2.4.6.7. Case Sensitive Search Process
When performing a case sensitive search ($caseSensitive: true) where the text index is case insensitive, the $text operator:
First searches the text index for case insensitive and diacritic matches. Then, to return just the documents that match the case of the search terms, the $text query operation includes an additional stage to filter out the documents that do not match the specified case. For case sensitive search (i.e. $caseSensitive: true), if the suffix stem contains uppercase letters, the $text operator matches on the exact word.
Specifying $caseSensitive: true may impact performance.
§ 2.4.6.8. Diacritic Insensitivity
The $text operator defaults to the diacritic insensitivity of the text index:
The version 3 text index is diacritic insensitive. That is, the index does not distinguish between characters that contain diacritical marks and their non-marked counterpart, such as é, ê, and e. Earlier versions of the text index are diacritic sensitive. $diacriticSensitive Option To support diacritic sensitive text search against the version 3 text index, specify $diacriticSensitive: true.
Text searches against earlier versions of the text index are inherently diacritic sensitive and cannot be diacritic insensitive. As such, the $diacriticSensitive option for the $text operator has no effect with earlier versions of the text index.
§ 2.4.6.9. Diacritic Sensitive Search Process
To perform a diacritic sensitive text search ($diacriticSensitive: true) against a version 3 text index, the $text operator:
First searches the text index, which is diacritic insensitive. Then, to return just the documents that match the diacritic marked characters of the search terms, the $text query operation includes an additional stage to filter out the documents that do not match. Specifying $diacriticSensitive: true may impact performance.
To perform a diacritic sensitive search against an earlier version of the text index, the $text operator searches the text index which is diacritic sensitive.
For diacritic sensitive search, if the suffix stem contains the diacritic mark or marks, the $text operator matches on the exact word.
§ 2.4.6.10. Text Score
The $text operator assigns a score to each document that contains the search term in the indexed fields. The score represents the relevance of a document to a given text search query. The score can be part of a sort() method specification as well as part of the projection expression. The { $meta: "textScore" } expression provides information on the processing of the $text operation. See $meta projection operator for details on accessing the score for projection or sort.
§ 2.4.6.11. Examples
Populate the collection with the following documents:
tim.X("user").New(
[
{ \_id: 1, subject: "coffee", author: "xyz", views: 50 },
{ \_id: 2, subject: "Coffee Shopping", author: "efg", views: 5 },
{ \_id: 3, subject: "Baking a cake", author: "abc", views: 90 },
{ \_id: 4, subject: "baking", author: "xyz", views: 100 },
{ \_id: 5, subject: "Café Con Leche", author: "abc", views: 200 },
{ \_id: 6, subject: "Сырники", author: "jkl", views: 80 },
{ \_id: 7, subject: "coffee and cream", author: "efg", views: 10 },
{ \_id: 8, subject: "Cafe con Leche", author: "xyz", views: 10 }
]
)
§ 2.4.6.12. Search for a Single Word
The following query specifies a $search string of coffee:
tim.X("user").Get( { $text: { $search: "coffee" } } )
This query returns the documents that contain the term coffee in the indexed subject field, or more precisely, the stemmed version of the word:
{ "\_id" : 2, "subject" : "Coffee Shopping", "author" : "efg", "views" : 5 }
{ "\_id" : 7, "subject" : "coffee and cream", "author" : "efg", "views" : 10 }
{ "\_id" : 1, "subject" : "coffee", "author" : "xyz", "views" : 50 }
§ 2.4.6.13. Match Any of the Search Terms
If the search string is a space-delimited string, $text operator performs a logical OR search on each term and returns documents that contains any of the terms.
The following query specifies a $search string of three terms delimited by space, "bake coffee cake":
tim.X("user").Get( { $text: { $search: "bake coffee cake" } } )
This query returns documents that contain either bake or coffee or cake in the indexed subject field, or more precisely, the stemmed version of these words:
{ "\_id" : 2, "subject" : "Coffee Shopping", "author" : "efg", "views" : 5 }
{ "\_id" : 7, "subject" : "coffee and cream", "author" : "efg", "views" : 10 }
{ "\_id" : 1, "subject" : "coffee", "author" : "xyz", "views" : 50 }
{ "\_id" : 3, "subject" : "Baking a cake", "author" : "abc", "views" : 90 }
{ "\_id" : 4, "subject" : "baking", "author" : "xyz", "views" : 100 }
§ 2.4.6.14. Search for a Phrase
To match the exact phrase as a single term, escape the quotes.
The following query searches for the phrase coffee shop:
tim.X("user").Get( { $text: { $search: "\"coffee shop\"" } } )
This query returns documents that contain the phrase coffee shop:
{ "\_id" : 2, "subject" : "Coffee Shopping", "author" : "efg", "views" : 5 }
§ 2.4.6.15. Exclude Documents That Contain a Term
A negated term is a term that is prefixed by a minus sign -. If you negate a term, the $text operator will exclude the documents that contain those terms from the results.
The following example searches for documents that contain the words coffee but do not contain the term shop, or more precisely the stemmed version of the words:
tim.X("user").Get( { $text: { $search: "coffee -shop" } } )
The query returns the following documents:
{ "\_id" : 7, "subject" : "coffee and cream", "author" : "efg", "views" : 10 }
{ "\_id" : 1, "subject" : "coffee", "author" : "xyz", "views" : 50 }
§ 2.4.6.16. Search a Different Language
Use the optional $language field in the $text expression to specify a language that determines the list of stop words and the rules for the stemmer and tokenizer for the search string.
If you specify a language value of "none", then the text search uses simple tokenization with no list of stop words and no stemming.
The following query specifies es, i.e. Spanish, as the language that determines the tokenization, stemming, and stop words:
tim.X("user").Get(
{ $text: { $search: "leche", $language: "es" } }
)
The query returns the following documents:
{ "\_id" : 5, "subject" : "Café Con Leche", "author" : "abc", "views" : 200 }
{ "\_id" : 8, "subject" : "Cafe con Leche", "author" : "xyz", "views" : 10 }
The $text expression can also accept the language by name, spanish. See Text Search Languages for the supported languages.
§ 2.4.6.17. Case and Diacritic Insensitive Search
The $text operator defers to the case and diacritic insensitivity of the text index. The version 3 text index is diacritic insensitive and expands its case insensitivity to include the Cyrillic alphabet as well as characters with diacritics. For details, see text Index Case Insensitivity and text Index Diacritic Insensitivity.
The following query performs a case and diacritic insensitive text search for the terms сы́рники or CAFÉS:
tim.X("user").Get( { $text: { $search: "сы́рники CAFÉS" } } )
Using the version 3 text index, the query matches the following documents.
{ "\_id" : 6, "subject" : "Сырники", "author" : "jkl", "views" : 80 }
{ "\_id" : 5, "subject" : "Café Con Leche", "author" : "abc", "views" : 200 }
{ "\_id" : 8, "subject" : "Cafe con Leche", "author" : "xyz", "views" : 10 }
With the previous versions of the text index, the query would not match any document.
§ 2.4.6.18. Perform Case Sensitive Search
To enable case sensitive search, specify $caseSensitive: true. Specifying $caseSensitive: true may impact performance.
1. Case Sensitive Search for a Term
The following query performs a case sensitive search for the term Coffee:
tim.X("user").Get( { $text: { $search: "Coffee", $caseSensitive: true } } )
The search matches just the document:
{ "\_id" : 2, "subject" : "Coffee Shopping", "author" : "efg", "views" : 5 }
2. Case Sensitive Search for a Phrase
The following query performs a case sensitive search for the phrase Café Con Leche:
tim.X("user").Get( {
$text: { $search: "\"Café Con Leche\"", $caseSensitive: true }
} )
The search matches just the document:
{ "\_id" : 5, "subject" : "Café Con Leche", "author" : "abc", "views" : 200 }
3. Case Sensitivity with Negated Term
A negated term is a term that is prefixed by a minus sign -. If you negate a term, the $text operator will exclude the documents that contain those terms from the results. You can also specify case sensitivity for negated terms.
The following example performs a case sensitive search for documents that contain the word Coffee but do not contain the lower-case term shop, or more precisely the stemmed version of the words:
tim.X("user").Get( { $text: { $search: "Coffee -shop", $caseSensitive: true } } )
The query matches the following document:
{ "\_id" : 2, "subject" : "Coffee Shopping", "author" : "efg" }
§ 2.4.6.19. Diacritic Sensitive Search
To enable diacritic sensitive search against a version 3 text index, specify $diacriticSensitive: true. Specifying $diacriticSensitive: true may impact performance.
1. Diacritic Sensitive Search for a Term
The following query performs a diacritic sensitive text search on the term CAFÉ, or more precisely the stemmed version of the word:
tim.X("user").Get( { $text: { $search: "CAFÉ", $diacriticSensitive: true } } )
The query only matches the following document:
{ "\_id" : 5, "subject" : "Café Con Leche", "author" : "abc" }
2. Diacritic Sensitivity with Negated Term
The $diacriticSensitive option applies also to negated terms. A negated term is a term that is prefixed by a minus sign -. If you negate a term, the $text operator will exclude the documents that contain those terms from the results.
The following query performs a diacritic sensitive text search for document that contains the term leches but not the term cafés, or more precisely the stemmed version of the words:
tim.X("user").Get(
{ $text: { $search: "leches -cafés", $diacriticSensitive: true } }
)
The query matches the following document:
{ "\_id" : 8, "subject" : "Cafe con Leche", "author" : "xyz" }
3. Return the Text Search Score
The following query searches for the term cake and returns the score assigned to each matching document:
tim.X("user").Get(
{ $text: { $search: "cake" } },
{ score: { $meta: "textScore" } }
)
The returned document includes an additional field score that contains the document’s score associated with the text search.
4. Sort by Text Search Score
To sort by the text score, include the same $meta expression in both the projection document and the sort expression. [1] The following query searches for the term coffee and sorts the results by the descending score:
tim.X("user").Get(
{ $text: { $search: "coffee" } },
{ score: { $meta: "textScore" } }
).sort( { score: { $meta: "textScore" } } )
The query returns the matching documents sorted by descending score.
5. Return Top 2 Matching Documents
Use the limit() method in conjunction with a sort() to return the top n matching documents.
The following query searches for the term coffee and sorts the results by the descending score, limiting the results to the top two matching documents:
tim.X("user").Get(
{ $text: { $search: "coffee" } },
{ score: { $meta: "textScore" } }
).sort( { score: { $meta: "textScore" } } ).limit(2)
6. Text Search with Additional Query and Sort Expressions
The following query searches for documents where the author equals "xyz" and the indexed field subject contains the terms coffee or bake. The operation also specifies a sort order of ascending date, then descending text search score:
tim.X("user").Get(
{ author: "xyz", $text: { $search: "coffee bake" } },
{ score: { $meta: "textScore" } }
).sort( { date: 1, score: { $meta: "textScore" } } )
7. Text Search in the Aggregation Pipeline
The behavior and requirements of the $meta projection operator differ from that of the $meta aggregation operator. For details on the $meta aggregation operator, see the $meta aggregation operator reference page.
¶ 2.4.7. $where
§ 2.4.7.1. Definition
Use the $where operator to pass either a string containing a JavaScript expression or a full JavaScript function to the query system. The $where provides greater flexibility, but requires that the database processes the JavaScript expression or function for each document in the collection. Reference the document in the JavaScript expression or function using either this or obj .
IMPORTANT
The $expr operator allows the use of aggregation expressions within the query language. $expr is faster than $where because it does not execute JavaScript and should be preferred where possible.
§ 2.4.7.2. Behavior of Restrictions
map-reduce operations, the group command, and $where operator expressions cannot access certain global functions or properties, such as db, that are available.
The following JavaScript functions and properties are available to map-reduce operations, the group command, and $where operator expressions:
Available Properties
args
MaxKey
MinKey
assert()
BinData()
DBPointer()
DBRef()
doassert()
emit()
gc()
HexData()
hex\_md5()
isNumber()
isObject()
ISODate()
isString()
Available Functions
Map()
MD5()
NumberInt()
NumberLong()
ObjectId()
print()
printjson()
printjsononeline()
sleep()
Timestamp()
tojson()
tojsononeline()
tojsonObject()
UUID()
version()
elemMatch
Only apply the $where query operator to top-level documents. The $where query operator will not work inside a nested document, for instance, in an $elemMatch query.
§ 2.4.7.3. Considerations
Do not use global variables. $where evaluates JavaScript and cannot take advantage of indexes. Therefore, query performance improves when you express your query using the standard operators (e.g., $gt, $in). In general, you should use $where only when you can’t express your query using another operator. If you must use $where, try to include at least one other standard query operator to filter the result set. Using $where alone requires a collection scan. Using normal non-$where query statements provides the following performance advantages:
X-Server will evaluate non-$where components of query before $where statements. If the non-$where statements match no documents, X-Server will not perform any query evaluation using $where. The non-$where query statements may use an index.
§ 2.4.7.4. Example
Consider the following documents in the users collection:
{
\_id: 12378,
name: "Steve",
username: "steveisawesome",
first\_login: "2017-01-01"
}
{
\_id: 2,
name: "Anya",
username: "anya",
first\_login: "2001-02-02"
}
The following example uses $where and the hex_md5() JavaScript function to compare the value of the name field to an MD5 hash and returns any matching document.
tim.X("user").Get( { $where: function() {
return (hex\_md5(this.name) == "9b53e667f30cd329dca1ec9e6a83e994")
} } );
The operation returns the following result:
{
"\_id" : 2,
"name" : "Anya",
"username" : "anya",
"first\_login" : "2001-02-02"
}
小礼物刷一波,打赏作者
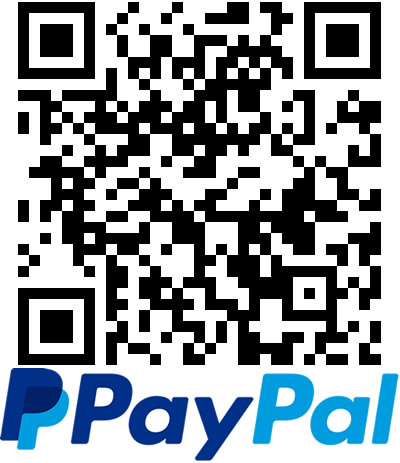
Paypal Donate
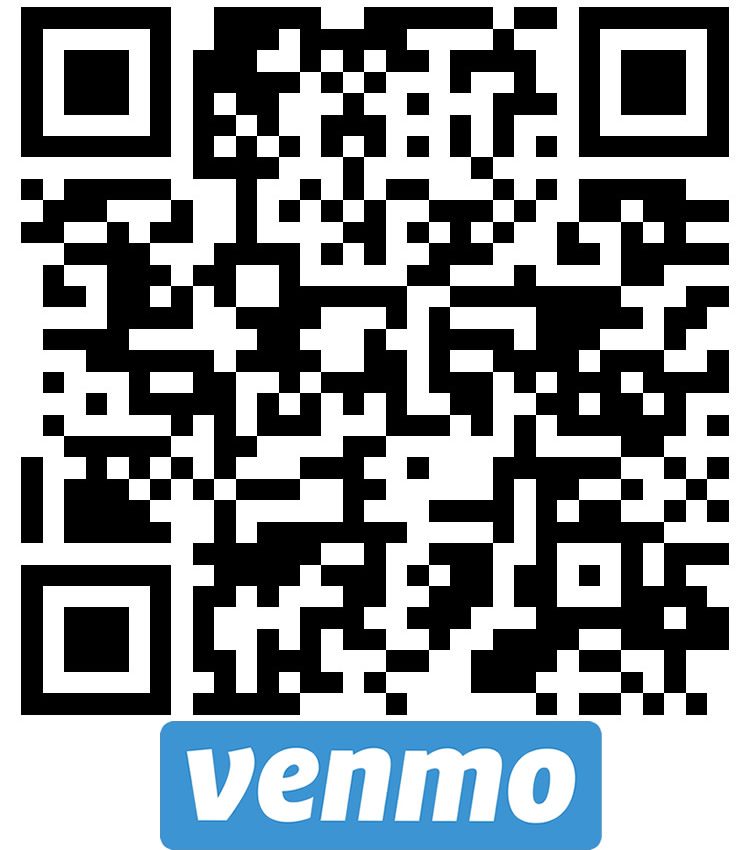
Venmo Donate
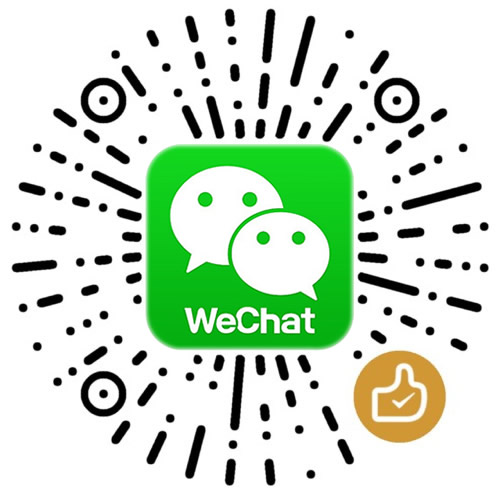
WeChat微信打赏
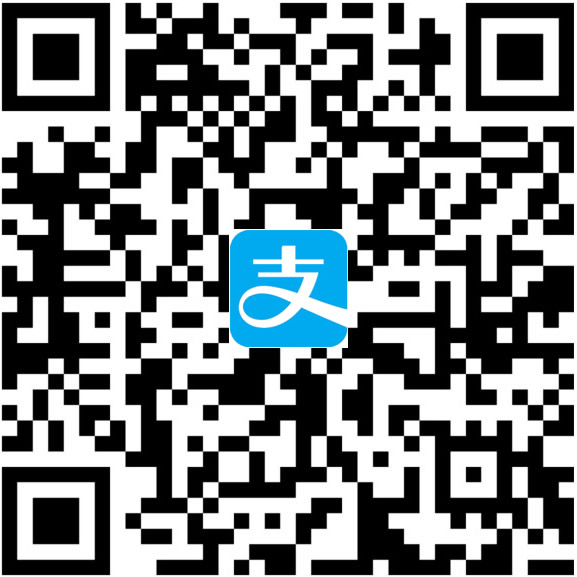
Alipay支付宝打赏